If a number remains same, even if we reverse its digits then the number is known as palindrome number. For example 12321 is a palindrome number because it remains same if we reverse its digits. In this article we have shared two C programs to check if the input number is palindrome or not. 1) using while loop 2) using recursion.
Program 1: check palindrome using while loop
/* Program to check if a number is palindrome or not
* using while loop
*/
#include <stdio.h>
int main()
{
int num, reverse_num=0, remainder,temp;
printf("Enter an integer: ");
scanf("%d", &num);
/* Here we are generating a new number (reverse_num)
* by reversing the digits of original input number
*/
temp=num;
while(temp!=0)
{
remainder=temp%10;
reverse_num=reverse_num*10+remainder;
temp/=10;
}
/* If the original input number (num) is equal to
* to its reverse (reverse_num) then its palindrome
* else it is not.
*/
if(reverse_num==num)
printf("%d is a palindrome number",num);
else
printf("%d is not a palindrome number",num);
return 0;
}
Output:
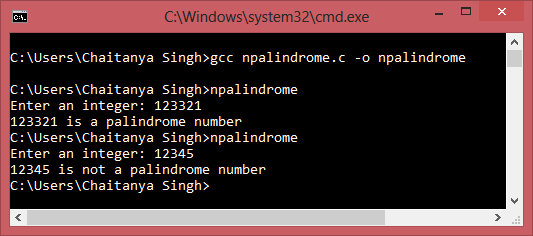
Program 2: check palindrome using recursion
#include<stdio.h>
int check_palindrome(int num){
static int reverse_num=0,rem;
if(num!=0){
rem=num%10;
reverse_num=reverse_num*10+rem;
check_palindrome(num/10);
}
return reverse_num;
}
int main(){
int num, reverse_num;
printf("Enter a number: ");
scanf("%d",&num);
reverse_num = check_palindrome(num);
if(num==reverse_num)
printf("%d is a palindrome number",num);
else
printf("%d is not a palindrome number",num);
return 0;
}
Output:
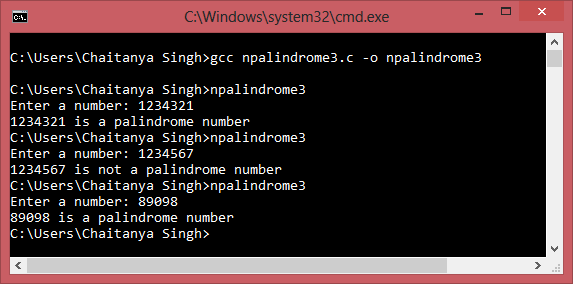
Comments
Post a Comment